Learn JS
JavaScript ("JS" for short) is a full-fledged dynamic programming language that, when applied to an HTML document, can provide dynamic interactivity on websites.
On Google Chrome you can access a JS console by doing Right-click -> Inspect -> Console
Callbacks
A callback is a function that is to be executed after another function has finished executing — hence the name ‘call back’. JavaScript is an event driven language. This means that instead of waiting for a response before moving on, JavaScript will keep executing while listening for other events.
What if function contains some sort of code that can’t be executed immediately? For example, an API request where we have to send the request then wait for a response? To simulate this action, were going to use setTimeout
which is a JavaScript function that calls a function after a set amount of time. We’ll delay our function for 500 milliseconds to simulate an API request. Our code will look like this:
function first() {
// Simulate a code delay
setTimeout(function() {
console.log(1);
}, 500);
}
function second() {
console.log(2);
}
first();
second();
So what happens now when we invoke our functions?
first();
second();
// 2
// 1
Selectors
To be able to change the UI and react to user events, we need to select HTML elements and modify them. For example we can get the title of a site by doing:
var myHeading = document.querySelector('h1');
querySelector
uses CSS selectors and is the most versatile of the JS selectors. JS also provides getElementById
, getElementsByClassName
and others.
Events
Real interactivity on a website needs events. These are code structures which listen for things happening in the browser, running code in response. The most obvious example is the click
event, which is fired by the browser when you click on something with your mouse.
document.querySelector('html').addEventListener('click', function() {
alert('Ouch! Stop poking me!');
})
Exercise 1
We will implement a wishlist. Please implement the UI showed in the images below. The user is able to see a list of all the items she/he wants to buy in the future. By pressing the "ADD" button the user will have a form to add new items to the list.
The user can also optimize which items to buy with a limited budget. When the user presses the "OPTIMIZE" button, the whishlist should be sorted from lowest cost to largest and the greatest number of items should be checked based on the budget.
Restriction: You can only use up to two iterations to optimize the wishlist.
Please use only plain JS (no libraries) in this exercise.
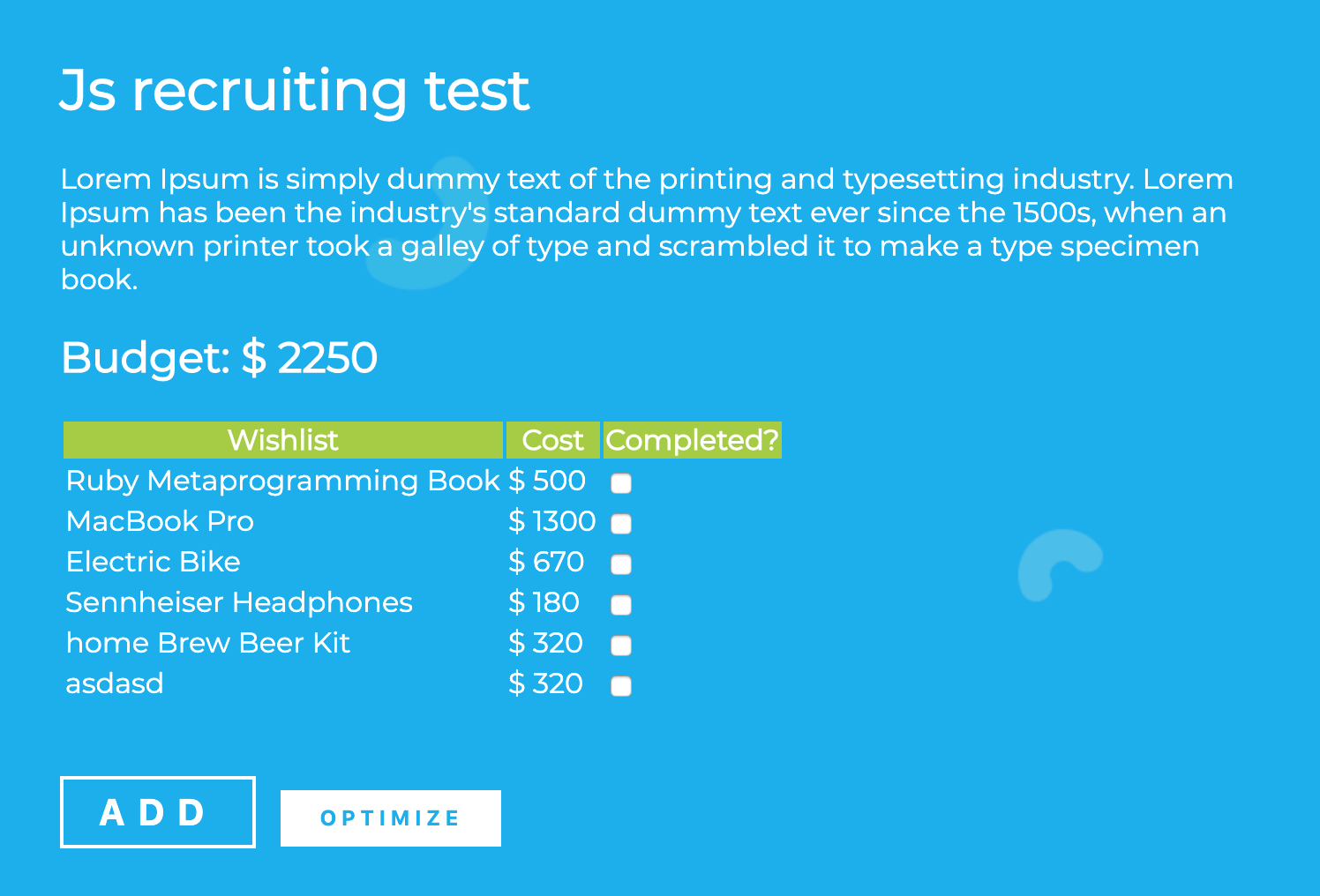
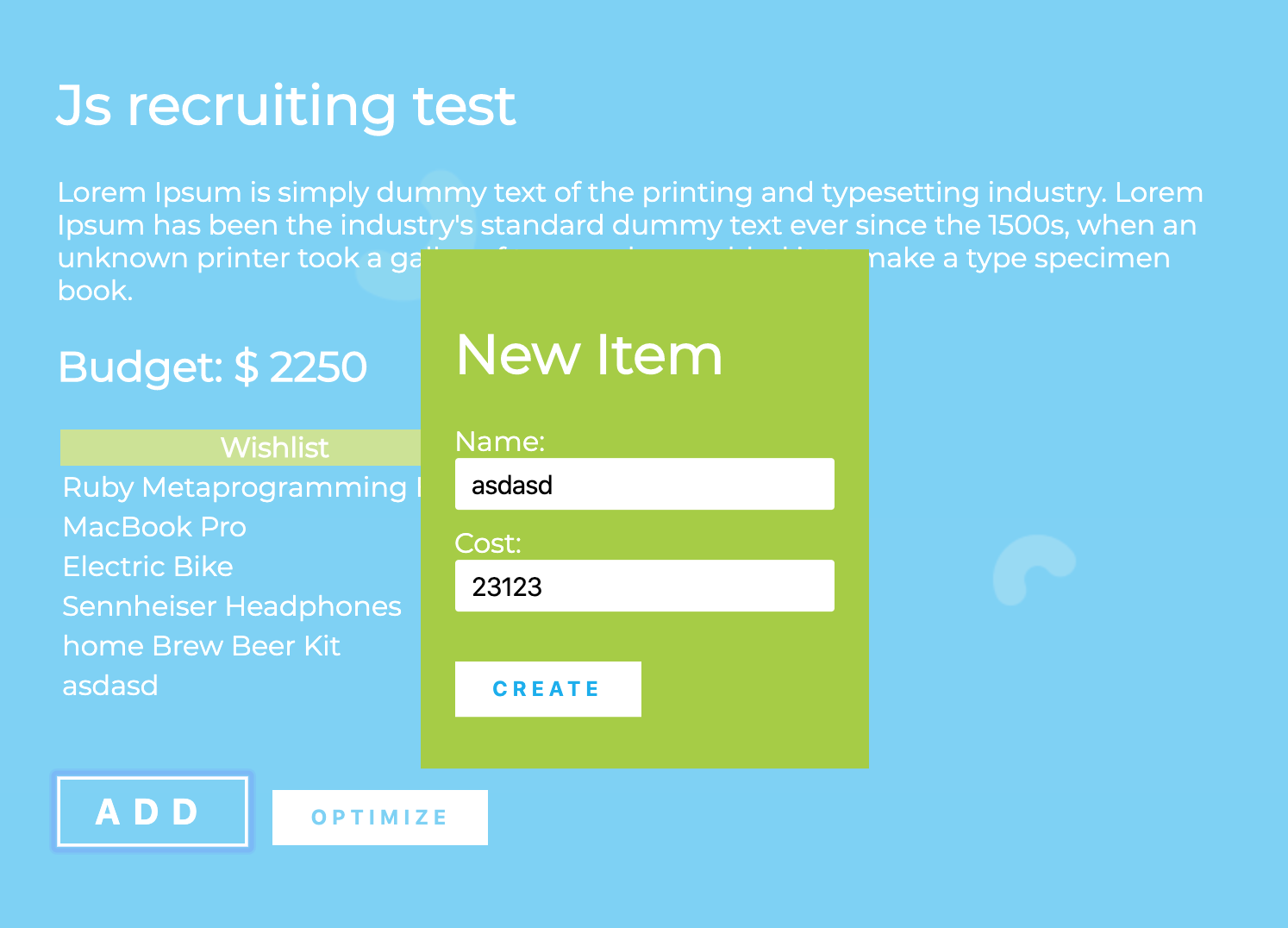
Exercise 2
We don't want to lose all items when the page is refreshed. To prevent this, when the user creates a new item, we need to persist it using localStorage
. Then, we will populate the wishlist with the previously stored items.
Additional Resources
- How to correctly name variables
- Things you SHOULDN'T do
- If you want to go deep into Javascript, check this javascript tutorial